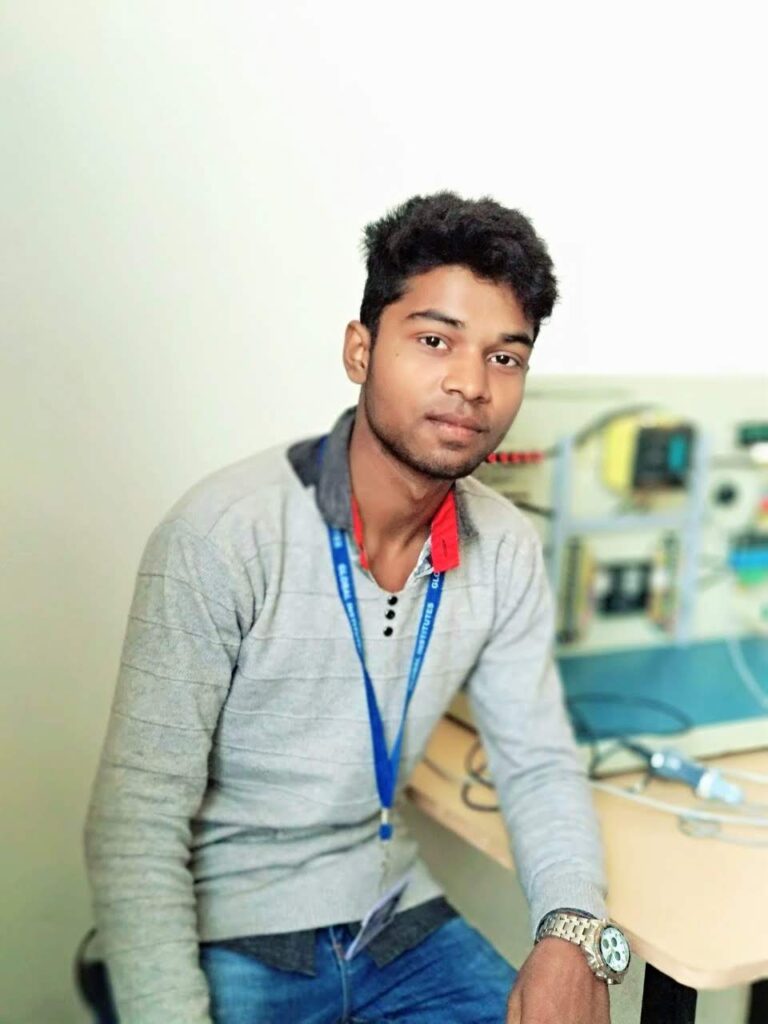
Mastering Java completely is quite challenging due to its vastness and the numerous field-specific libraries. However, you can definitely get a solid grasp of its syntax.
Understanding Java’s Unique Features
Java stands out from C and C++ primarily as an object-oriented programming (OOP) language. This means it allows you to model real-world concepts, such as inheritance. One of its key features is “Write Once, Run Anywhere” (WORA), meaning that compiled Java code can run on any platform (Windows, Linux, Mac OS, etc.). This is made possible because Java code is compiled into bytecode, which is platform-independent, and this bytecode is executed by the Java Virtual Machine (JVM), which is specific to each platform. For more in-depth knowledge, consider reading “Java: The Complete Reference” by Herbert Schildt.
Getting Started
Once you understand the foundational concepts, begin practicing with simple programs to familiarize yourself with Java’s syntax. Remember, Java is strongly typed and case-sensitive, often using CamelCase notation. It’s essential to grasp the significance of keywords like “class,” “public,” “static,” “void,” and “main,” as omitting any can affect your code. For beginners, I recommend using BlueJ IDE, which is user-friendly and perfect for learning.
Input Handling
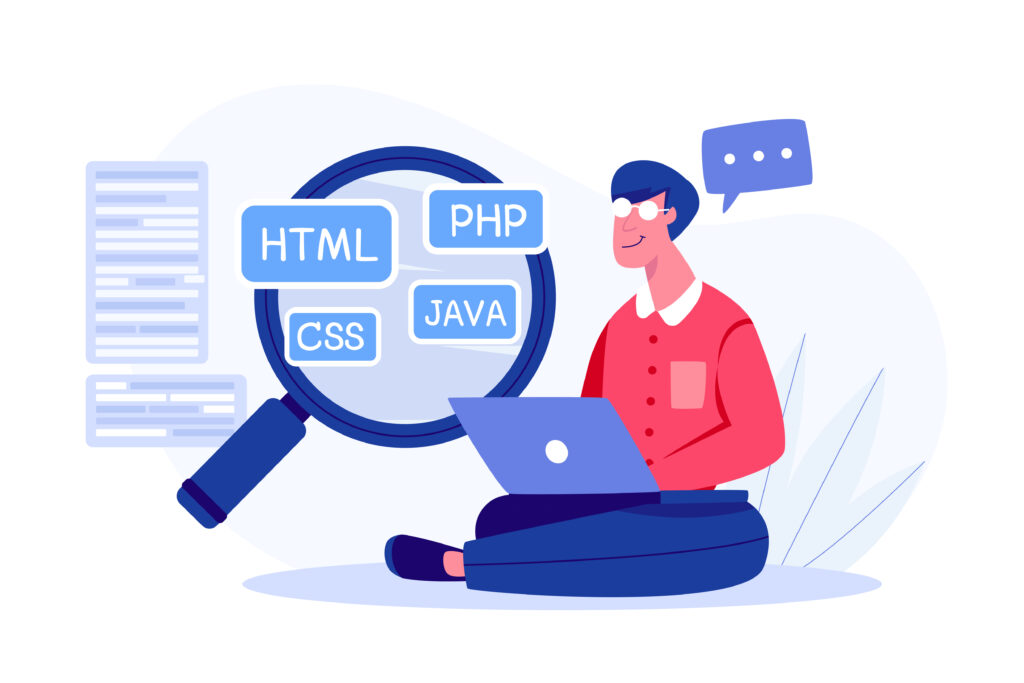
Once you’re comfortable with the basics, learn to accept user input via the command line. Familiarize yourself with the Scanner
and BufferedReader
classes; while I prefer BufferedReader
for its robustness, it can be a bit more complex.
Control Structures
Next, dive into loops: for
, while
, do-while
, and enhanced for
loops.
Functions and Recursion
Understanding functions and recursion is crucial, as functions will transition into methods when working with classes and objects. Focus on building a strong foundation in functions.
Variables and Memory Management
Learn about static versus non-static variables, and understand how Java handles passing arguments by value rather than by reference (unlike C/C++, Java does not use pointers).
Strings and Arrays
After functions, explore strings. In Java, strings are treated as objects, which distinguishes them from character arrays in C/C++. Understanding StringBuffer
, StringBuilder
, and the String
class is important for efficient memory management. Then, familiarize yourself with arrays, both static and dynamic, and practice sorting and searching algorithms for better comprehension.
Object-Oriented Concepts
Once you’re comfortable with these topics, study access modifiers: “public,” “protected,” “private,” and “static.” These are vital for OOP principles. Learn about packages and their significance in Java.
Start programming with classes and objects. Create a few classes and practice accessing each other’s members using objects. Experiment with access modifiers and explore inheritance and encapsulation concepts.
Core Java Libraries
After mastering the fundamentals, familiarize yourself with useful built-in classes like ArrayList
, List
, HashMap
, and HashSet
, and study generic classes.
These topics form the core of Java, and many companies assess your understanding of these areas. Consider preparing for an Oracle Certification exam; with diligent study and practice, it can significantly enhance your resume.
Specialization
Once you have a solid foundation, choose a specialization, such as web development, Android development, or data analytics. You can explore connecting databases, sending data via TCP/UDP, and working with Servlets and JSP. The field is expansive!
Resources
I hope this gives you a clear path forward. For online tutorials, platforms like Udemy, TutorialsPoint, and Codecademy can be helpful. The more resources you engage with, the stronger your understanding will become. Don’t forget to check out GeeksforGeeks as well!
Let me know if you need any more adjustments or additional information!